React: Zoom In & Out with the Mouse Wheel
Listen to [Ctrl] + Scroll Wheel Event
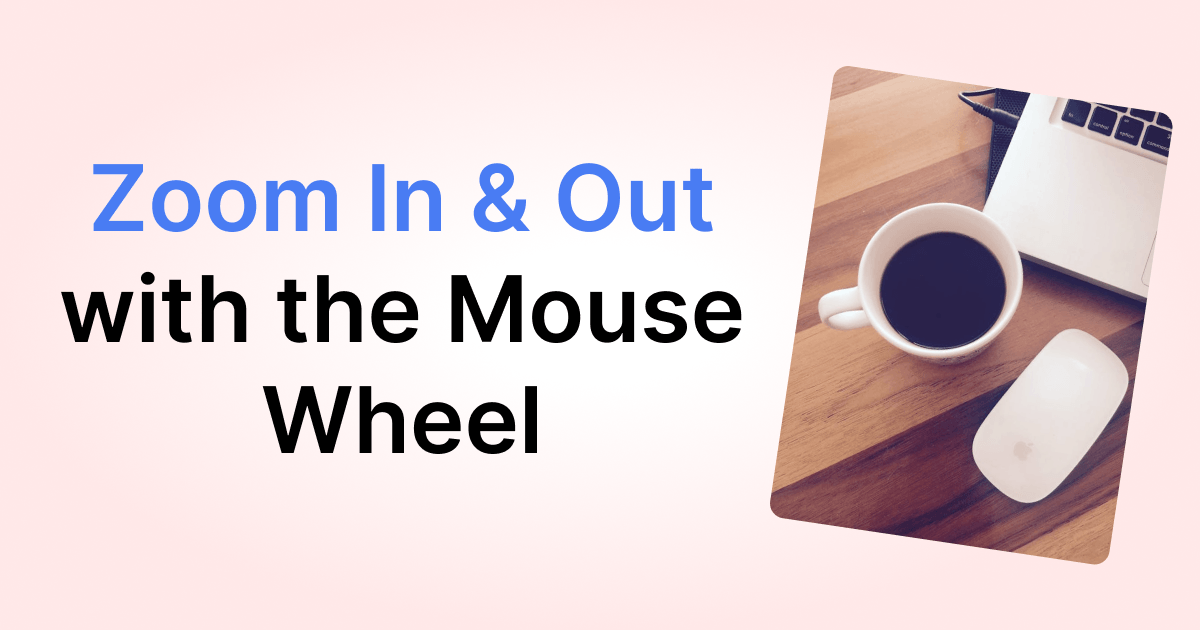
Do you want to zoom in and out your React UI by holding the Ctrl (control) key and the mouse wheel? Let’s take a look at how we can easily archive this.
React has the well-known onClick
event handler. It has for the mousewheel the onWheel
handler. But you might see the error:
Unable to preventdefault inside passive event listener invocation
Instead, you assign a proper event listener:
"use client"
import { useEffect } from "react"
export const Editor = () => {
useEffect(() => {
const handleWheel = (e: WheelEvent) => {
// Windows & Linux: ctrl, Mac: meta
if (e.ctrlKey || e.metaKey) {
e.preventDefault()
console.log(`mousewheel ${e.deltaY < 0 ? "zoom out" : "zoom in"}`)
}
}
document.addEventListener("wheel", handleWheel, { passive: false })
return () => {
// Remove listener if component is removed
document.removeEventListener("wheel", handleWheel)
}
}, [])
return (<>{/* My components here */}</>)
}
Article Changes
- June 20, 2024: Added
removeEventListener
andmetaKey
to code snippet.